mirror of
https://github.com/project-redbud/FunGame-Server.git
synced 2025-04-22 03:59:36 +08:00
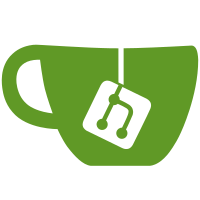
* 添加 Web API 和 RESTful API 模式; * 添加 SQLite 模式; * 添加 ISocketMessageProcessor 和 ISocketListener<> 接口,用于统一数据访问; * 重做了 ISocketModel; * 完善了 WebSocket 的连接模式。
59 lines
2.2 KiB
C#
59 lines
2.2 KiB
C#
using Microsoft.AspNetCore.Authorization;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
using Milimoe.FunGame.Core.Api.Utility;
|
|
using Milimoe.FunGame.Core.Library.Common.Network;
|
|
using Milimoe.FunGame.Core.Library.Constant;
|
|
using Milimoe.FunGame.WebAPI.Architecture;
|
|
using Milimoe.FunGame.WebAPI.Models;
|
|
|
|
namespace Milimoe.FunGame.WebAPI.Controllers
|
|
{
|
|
[ApiController]
|
|
[Route("[controller]")]
|
|
[Authorize]
|
|
public class PostDataController(ILogger<PostDataController> logger) : ControllerBase
|
|
{
|
|
public static Dictionary<Guid, SocketObject> ResultDatas { get; } = [];
|
|
|
|
private readonly ILogger<PostDataController> _logger = logger;
|
|
|
|
[HttpPost("{username}", Name = "username")]
|
|
public async Task<IActionResult> Post(string username, [FromBody] SocketObject obj)
|
|
{
|
|
try
|
|
{
|
|
RESTfulAPIListener? apiListener = Singleton.Get<RESTfulAPIListener>();
|
|
if (apiListener != null && apiListener.UserList.ContainsKey(username))
|
|
{
|
|
RESTfulAPIModel model = (RESTfulAPIModel)apiListener.UserList[username];
|
|
if (model.LastRequestID == Guid.Empty)
|
|
{
|
|
Guid uid = Guid.NewGuid();
|
|
model.LastRequestID = uid;
|
|
await model.SocketMessageHandler(model.Socket, obj);
|
|
model.LastRequestID = Guid.Empty;
|
|
if (ResultDatas.TryGetValue(uid, out SocketObject list))
|
|
{
|
|
return Ok(list);
|
|
}
|
|
else
|
|
{
|
|
return NotFound();
|
|
}
|
|
}
|
|
else
|
|
{
|
|
Ok(new SocketObject(SocketMessageType.System, model.Token, "请求未执行完毕,请等待!"));
|
|
}
|
|
}
|
|
return NotFound();
|
|
}
|
|
catch (Exception e)
|
|
{
|
|
_logger.LogError(e, "Error during post data");
|
|
return StatusCode(500, "服务器内部错误");
|
|
}
|
|
}
|
|
}
|
|
}
|