mirror of
https://github.com/project-redbud/FunGame-Core.git
synced 2025-04-22 03:59:35 +08:00
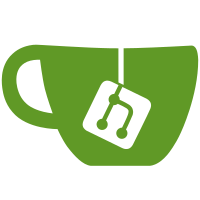
* 重做 WebSocket 监听;为服务器统一了多种数据连接访问时的处理;统一编码为 UTF-8 * ModelManager已更名并移动到工具命名空间中 * 完成 WebSocket 消息处理系统 * 添加Socket异步接收数据流;修复TaskUtility阻塞的问题;优化心跳、房间、模组 * 添加枚举 * 删除多余字符 * 添加监听器的名称 * 修改了命名
108 lines
3.5 KiB
C#
108 lines
3.5 KiB
C#
using Milimoe.FunGame.Core.Interface.Sockets;
|
|
using Milimoe.FunGame.Core.Library.Common.Architecture;
|
|
using Milimoe.FunGame.Core.Library.Constant;
|
|
using Milimoe.FunGame.Core.Library.Exception;
|
|
using Milimoe.FunGame.Core.Service;
|
|
|
|
namespace Milimoe.FunGame.Core.Library.Common.Network
|
|
{
|
|
public class Socket : IClientSocket
|
|
{
|
|
public System.Net.Sockets.Socket Instance { get; }
|
|
public SocketRuntimeType Runtime => SocketRuntimeType.Client;
|
|
public Guid Token { get; set; } = Guid.Empty;
|
|
public string ServerAddress { get; } = "";
|
|
public int ServerPort { get; } = 0;
|
|
public string ServerName { get; } = "";
|
|
public string ServerNotice { get; } = "";
|
|
public bool Connected => Instance != null && Instance.Connected;
|
|
public bool Receiving => _receiving;
|
|
private HeartBeat HeartBeat { get; }
|
|
|
|
private Task? _receivingTask;
|
|
private bool _receiving = false;
|
|
private readonly HashSet<Action<SocketObject>> _boundEvents = [];
|
|
|
|
private Socket(System.Net.Sockets.Socket instance, string serverAddress, int serverPort)
|
|
{
|
|
this.Instance = instance;
|
|
this.ServerAddress = serverAddress;
|
|
this.ServerPort = serverPort;
|
|
HeartBeat = new(this);
|
|
HeartBeat.StartSendingHeartBeat();
|
|
}
|
|
|
|
public static Socket Connect(string address, int port = 22222)
|
|
{
|
|
System.Net.Sockets.Socket? socket = SocketManager.Connect(address, port);
|
|
if (socket != null) return new Socket(socket, address, port);
|
|
else throw new ConnectFailedException();
|
|
}
|
|
|
|
public SocketResult Send(SocketMessageType type, params object[] objs)
|
|
{
|
|
if (Instance != null)
|
|
{
|
|
if (SocketManager.Send(new(type, Token, objs)) == SocketResult.Success)
|
|
{
|
|
return SocketResult.Success;
|
|
}
|
|
return SocketResult.Fail;
|
|
}
|
|
return SocketResult.NotSent;
|
|
}
|
|
|
|
public SocketObject[] Receive()
|
|
{
|
|
try
|
|
{
|
|
SocketObject[] result = SocketManager.Receive();
|
|
return result;
|
|
}
|
|
catch (System.Exception e)
|
|
{
|
|
Api.Utility.TXTHelper.AppendErrorLog(e.GetErrorInfo());
|
|
throw new SocketWrongInfoException();
|
|
}
|
|
}
|
|
|
|
public void AddSocketObjectHandler(Action<SocketObject> method)
|
|
{
|
|
if (_boundEvents.Add(method))
|
|
{
|
|
SocketManager.SocketReceive += new SocketManager.SocketReceiveHandler(method);
|
|
}
|
|
}
|
|
|
|
public void RemoveSocketObjectHandler(Action<SocketObject> method)
|
|
{
|
|
_boundEvents.Remove(method);
|
|
SocketManager.SocketReceive -= new SocketManager.SocketReceiveHandler(method);
|
|
}
|
|
|
|
public void Close()
|
|
{
|
|
HeartBeat.StopSendingHeartBeat();
|
|
StopReceiving();
|
|
Instance?.Close();
|
|
foreach (Action<SocketObject> method in _boundEvents.ToList())
|
|
{
|
|
RemoveSocketObjectHandler(method);
|
|
}
|
|
}
|
|
|
|
public void StartReceiving(Task t)
|
|
{
|
|
_receiving = true;
|
|
_receivingTask = t;
|
|
}
|
|
|
|
public void StopReceiving()
|
|
{
|
|
_receiving = false;
|
|
_receivingTask?.Wait(1);
|
|
_receivingTask = null;
|
|
}
|
|
}
|
|
}
|