mirror of
https://github.com/project-redbud/FunGame-Core.git
synced 2025-04-22 03:59:35 +08:00
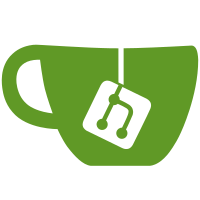
* 添加大量新的枚举,添加缺少的枚举字符串,修改枚举转字符串方法的位置 * 添加StartGame和Gaming的SocketHandler方法 * 添加MainInvokeType.StartGame * 优化代码格式 * 添加默认的User.ToString() * 添加EndGame * 添加GameMode.GetTypeString * 添加GameMode.GetRoomType
74 lines
2.7 KiB
C#
74 lines
2.7 KiB
C#
using System.Data;
|
|
using Milimoe.FunGame.Core.Api.Transmittal;
|
|
using Milimoe.FunGame.Core.Library.Constant;
|
|
using Milimoe.FunGame.Core.Library.SQLScript.Entity;
|
|
|
|
namespace Milimoe.FunGame.Core.Library.Common.Architecture
|
|
{
|
|
public abstract class Authenticator
|
|
{
|
|
private readonly SQLHelper SQLHelper;
|
|
|
|
public Authenticator(SQLHelper SQLHelper)
|
|
{
|
|
this.SQLHelper = SQLHelper;
|
|
}
|
|
|
|
public abstract bool BeforeAuthenticator(AuthenticationType type, params object[] args);
|
|
|
|
public abstract bool AfterAuthenticator(AuthenticationType type, params object[] args);
|
|
|
|
public bool Authenticate(string script)
|
|
{
|
|
if (!BeforeAuthenticator(AuthenticationType.ScriptOnly, script)) return false;
|
|
SQLHelper.ExecuteDataSet(script);
|
|
if (SQLHelper.Success)
|
|
{
|
|
DataSet ds = SQLHelper.DataSet;
|
|
if (ds.Tables.Count > 0 &&
|
|
ds.Tables[0].Rows.Count > 0)
|
|
{
|
|
if (!AfterAuthenticator(AuthenticationType.ScriptOnly, script)) return false;
|
|
return true;
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
|
|
public bool Authenticate<T>(string script, string column, T keyword)
|
|
{
|
|
if (!BeforeAuthenticator(AuthenticationType.Column, script, column, keyword?.ToString() ?? "")) return false;
|
|
SQLHelper.ExecuteDataSet(script);
|
|
if (SQLHelper.Success)
|
|
{
|
|
DataSet ds = SQLHelper.DataSet;
|
|
if (ds.Tables.Count > 0 &&
|
|
ds.Tables[0].Columns.Contains(column) &&
|
|
ds.Tables[0].Rows.Count > 0 &&
|
|
ds.Tables[0].AsEnumerable().Where(row => row.Field<T>(column)?.Equals(keyword) ?? false).Any())
|
|
{
|
|
if (!AfterAuthenticator(AuthenticationType.Column, script, column, keyword?.ToString() ?? "")) return false;
|
|
return true;
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
|
|
public bool Authenticate(string username, string password)
|
|
{
|
|
if (!BeforeAuthenticator(AuthenticationType.Username, username, password)) return false;
|
|
SQLHelper.ExecuteDataSet(UserQuery.Select_Users_LoginQuery(username, password));
|
|
if (SQLHelper.Success)
|
|
{
|
|
DataSet ds = SQLHelper.DataSet;
|
|
if (ds.Tables.Count > 0 && ds.Tables[0].Rows.Count > 0)
|
|
{
|
|
if (!AfterAuthenticator(AuthenticationType.Username, username, password)) return false;
|
|
return true;
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
}
|
|
}
|