mirror of
https://github.com/project-redbud/FunGame-Core.git
synced 2025-04-21 19:49:34 +08:00
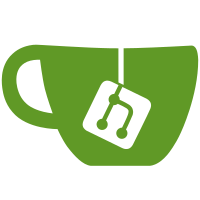
* 添加 OpenFactory,可以动态扩展技能和物品 * 修改 Effect 的反序列化解析;增加对闪避/暴击判定的先前事件编程接口 * 补充魔法伤害的判定 * 装备系统优化;角色的复制问题修复 * 添加物品品质;更新装备饰品替换机制;添加第一滴血、团队模式 * 添加技能选取 * 添加团队死斗模式
90 lines
2.4 KiB
C#
90 lines
2.4 KiB
C#
using Milimoe.FunGame.Core.Api.Utility;
|
||
using Milimoe.FunGame.Core.Entity;
|
||
using Milimoe.FunGame.Core.Interface.Addons;
|
||
|
||
namespace Milimoe.FunGame.Core.Library.Common.Addon
|
||
{
|
||
public abstract class SkillModule : IAddon
|
||
{
|
||
/// <summary>
|
||
/// 模组名称
|
||
/// </summary>
|
||
public abstract string Name { get; }
|
||
|
||
/// <summary>
|
||
/// 模组描述
|
||
/// </summary>
|
||
public abstract string Description { get; }
|
||
|
||
/// <summary>
|
||
/// 模组版本
|
||
/// </summary>
|
||
public abstract string Version { get; }
|
||
|
||
/// <summary>
|
||
/// 模组作者
|
||
/// </summary>
|
||
public abstract string Author { get; }
|
||
|
||
/// <summary>
|
||
/// 此模组中包含的技能
|
||
/// </summary>
|
||
public abstract Dictionary<string, Skill> Skills { get; }
|
||
|
||
/// <summary>
|
||
/// 加载标记
|
||
/// </summary>
|
||
private bool IsLoaded = false;
|
||
|
||
/// <summary>
|
||
/// 加载模组
|
||
/// </summary>
|
||
public bool Load(params object[] objs)
|
||
{
|
||
if (IsLoaded)
|
||
{
|
||
return false;
|
||
}
|
||
// BeforeLoad可以阻止加载此模组
|
||
if (BeforeLoad())
|
||
{
|
||
// 模组加载后,不允许再次加载此模组
|
||
IsLoaded = true;
|
||
// 注册工厂
|
||
Factory.OpenFactory.RegisterFactory(SkillFactory());
|
||
Factory.OpenFactory.RegisterFactory(EffectFactory());
|
||
// 如果加载后需要执行代码,请重写AfterLoad方法
|
||
AfterLoad();
|
||
}
|
||
return IsLoaded;
|
||
}
|
||
|
||
/// <summary>
|
||
/// 注册工厂
|
||
/// </summary>
|
||
protected abstract Factory.EntityFactoryDelegate<Skill> SkillFactory();
|
||
|
||
/// <summary>
|
||
/// 注册工厂(特效类)
|
||
/// </summary>
|
||
protected abstract Factory.EntityFactoryDelegate<Effect> EffectFactory();
|
||
|
||
/// <summary>
|
||
/// 模组加载后需要做的事
|
||
/// </summary>
|
||
protected virtual void AfterLoad()
|
||
{
|
||
// override
|
||
}
|
||
|
||
/// <summary>
|
||
/// 允许返回false来阻止加载此模组
|
||
/// </summary>
|
||
/// <returns></returns>
|
||
protected virtual bool BeforeLoad()
|
||
{
|
||
return true;
|
||
}
|
||
}
|
||
}
|