mirror of
https://github.com/project-redbud/FunGame-Core.git
synced 2025-04-22 03:59:35 +08:00
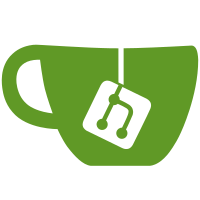
* 使基于HTTPClient的DataRequest能够收到回复;添加了适用于Gaming的DataRequest;优化了加载器的加载逻辑;依赖集合的优化 * 执行代理清理;优化模组模板 * 删除GamingEvent无用的事件;删除result哈希表;删除无用的Item/Skill类;GameModuleLoader优化
101 lines
3.1 KiB
C#
101 lines
3.1 KiB
C#
using Milimoe.FunGame.Core.Interface.Sockets;
|
|
using Milimoe.FunGame.Core.Library.Common.Architecture;
|
|
using Milimoe.FunGame.Core.Library.Constant;
|
|
using Milimoe.FunGame.Core.Library.Exception;
|
|
using Milimoe.FunGame.Core.Service;
|
|
|
|
namespace Milimoe.FunGame.Core.Library.Common.Network
|
|
{
|
|
public class Socket : IClientSocket
|
|
{
|
|
public System.Net.Sockets.Socket Instance { get; }
|
|
public SocketRuntimeType Runtime => SocketRuntimeType.Client;
|
|
public Guid Token { get; set; } = Guid.Empty;
|
|
public string ServerAddress { get; } = "";
|
|
public int ServerPort { get; } = 0;
|
|
public string ServerName { get; } = "";
|
|
public string ServerNotice { get; } = "";
|
|
public bool Connected => Instance != null && Instance.Connected;
|
|
public bool Receiving => _Receiving;
|
|
|
|
private Task? ReceivingTask;
|
|
private readonly HeartBeat HeartBeat;
|
|
private bool _Receiving = false;
|
|
|
|
private Socket(System.Net.Sockets.Socket Instance, string ServerAddress, int ServerPort)
|
|
{
|
|
this.Instance = Instance;
|
|
this.ServerAddress = ServerAddress;
|
|
this.ServerPort = ServerPort;
|
|
HeartBeat = new(this);
|
|
HeartBeat.StartSendingHeartBeat();
|
|
}
|
|
|
|
public static Socket Connect(string Address, int Port = 22222)
|
|
{
|
|
System.Net.Sockets.Socket? socket = SocketManager.Connect(Address, Port);
|
|
if (socket != null) return new Socket(socket, Address, Port);
|
|
else throw new ConnectFailedException();
|
|
}
|
|
|
|
public SocketResult Send(SocketMessageType type, params object[] objs)
|
|
{
|
|
if (Instance != null)
|
|
{
|
|
if (SocketManager.Send(new(type, Token, objs)) == SocketResult.Success)
|
|
{
|
|
return SocketResult.Success;
|
|
}
|
|
return SocketResult.Fail;
|
|
}
|
|
return SocketResult.NotSent;
|
|
}
|
|
|
|
public SocketObject[] Receive()
|
|
{
|
|
try
|
|
{
|
|
SocketObject[] result = SocketManager.Receive();
|
|
return result;
|
|
}
|
|
catch (System.Exception e)
|
|
{
|
|
Api.Utility.TXTHelper.AppendErrorLog(e.GetErrorInfo());
|
|
throw new SocketWrongInfoException();
|
|
}
|
|
}
|
|
|
|
public void BindEvent(Delegate method, bool remove = false)
|
|
{
|
|
if (!remove)
|
|
{
|
|
SocketManager.SocketReceive += (SocketManager.SocketReceiveHandler)method;
|
|
}
|
|
else
|
|
{
|
|
SocketManager.SocketReceive -= (SocketManager.SocketReceiveHandler)method;
|
|
}
|
|
}
|
|
|
|
public void Close()
|
|
{
|
|
HeartBeat.StopSendingHeartBeat();
|
|
StopReceiving();
|
|
Instance?.Close();
|
|
}
|
|
|
|
public void StartReceiving(Task t)
|
|
{
|
|
_Receiving = true;
|
|
ReceivingTask = t;
|
|
}
|
|
|
|
private void StopReceiving()
|
|
{
|
|
_Receiving = false;
|
|
ReceivingTask?.Wait(1);
|
|
ReceivingTask = null;
|
|
}
|
|
}
|
|
}
|