mirror of
https://github.com/project-redbud/FunGame-Core.git
synced 2025-04-22 12:09:34 +08:00
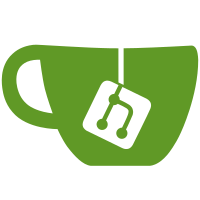
* 添加 OpenFactory,可以动态扩展技能和物品 * 修改 Effect 的反序列化解析;增加对闪避/暴击判定的先前事件编程接口 * 补充魔法伤害的判定 * 装备系统优化;角色的复制问题修复 * 添加物品品质;更新装备饰品替换机制;添加第一滴血、团队模式 * 添加技能选取 * 添加团队死斗模式
94 lines
3.7 KiB
C#
94 lines
3.7 KiB
C#
using Milimoe.FunGame.Core.Api.Utility;
|
|
using Milimoe.FunGame.Core.Entity;
|
|
|
|
namespace Milimoe.FunGame.Core.Library.Common.Addon
|
|
{
|
|
/// <summary>
|
|
/// 模组的依赖集合<para/>
|
|
/// <paramref name="maps"></paramref>(地图名称(<see cref="GameMap.Name"/>)的数组)<para/>
|
|
/// <paramref name="characters"></paramref>(角色模组名称(<see cref="CharacterModule.Name"/>)的数组)<para/>
|
|
/// <paramref name="skills"></paramref>(技能模组名称(<see cref="SkillModule.Name"/>)的数组)<para/>
|
|
/// <paramref name="items"></paramref>(物品模组名称(<see cref="ItemModule.Name"/>)的数组)
|
|
/// </summary>
|
|
public readonly struct GameModuleDepend(string[] maps, string[] characters, string[] skills, string[] items)
|
|
{
|
|
/// <summary>
|
|
/// 模组所使用的地图组
|
|
/// </summary>
|
|
public string[] MapsDepend { get; } = maps;
|
|
|
|
/// <summary>
|
|
/// 模组所使用的角色组
|
|
/// </summary>
|
|
public string[] CharactersDepend { get; } = characters;
|
|
|
|
/// <summary>
|
|
/// 模组所使用的技能组
|
|
/// </summary>
|
|
public string[] SkillsDepend { get; } = skills;
|
|
|
|
/// <summary>
|
|
/// 模组所使用的物品组
|
|
/// </summary>
|
|
public string[] ItemsDepend { get; } = items;
|
|
|
|
/// <summary>
|
|
/// 实际使用的地图组对象<para/>
|
|
/// 请使用 <see cref="GetDependencies"/> 自动填充,不要自己添加
|
|
/// </summary>
|
|
public List<GameMap> Maps { get; } = [];
|
|
|
|
/// <summary>
|
|
/// 实际使用的角色组对象<para/>
|
|
/// 请使用 <see cref="GetDependencies"/> 自动填充,不要自己添加
|
|
/// </summary>
|
|
public Dictionary<string, Character> Characters { get; } = [];
|
|
|
|
/// <summary>
|
|
/// 实际使用的技能组对象<para/>
|
|
/// 请使用 <see cref="GetDependencies"/> 自动填充,不要自己添加
|
|
/// </summary>
|
|
public Dictionary<string, Skill> Skills { get; } = [];
|
|
|
|
/// <summary>
|
|
/// 实际使用的物品组对象<para/>
|
|
/// 请使用 <see cref="GetDependencies"/> 自动填充,不要自己添加
|
|
/// </summary>
|
|
public Dictionary<string, Item> Items { get; } = [];
|
|
|
|
/// <summary>
|
|
/// 获得所有的依赖项<para/>
|
|
/// 此方法会自动填充 <see cref="Maps"/> <see cref="Characters"/> <see cref="Skills"/> <see cref="Items"/>
|
|
/// </summary>
|
|
public void GetDependencies(GameModuleLoader loader)
|
|
{
|
|
Maps.Clear();
|
|
Characters.Clear();
|
|
Skills.Clear();
|
|
Items.Clear();
|
|
Maps.AddRange(loader.Maps.Keys.Where(MapsDepend.Contains).Select(str => loader.Maps[str]));
|
|
foreach (CharacterModule modules in loader.Characters.Keys.Where(CharactersDepend.Contains).Select(str => loader.Characters[str]))
|
|
{
|
|
foreach (string key in modules.Characters.Keys)
|
|
{
|
|
Characters[key] = modules.Characters[key];
|
|
}
|
|
}
|
|
foreach (SkillModule modules in loader.Skills.Keys.Where(SkillsDepend.Contains).Select(str => loader.Skills[str]))
|
|
{
|
|
foreach (string key in modules.Skills.Keys)
|
|
{
|
|
Skills[key] = modules.Skills[key];
|
|
}
|
|
}
|
|
foreach (ItemModule modules in loader.Items.Keys.Where(ItemsDepend.Contains).Select(str => loader.Items[str]))
|
|
{
|
|
foreach (string key in modules.Items.Keys)
|
|
{
|
|
Items[key] = modules.Items[key];
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|