mirror of
https://github.com/project-redbud/FunGame-Core.git
synced 2025-04-21 03:29:36 +08:00
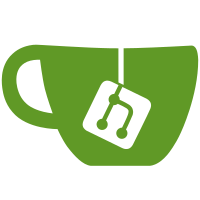
* 添加SQLite模式 * 将Hashtable转为Dictionary<string, object>,因为它具有性能优势 * 添加GamingRequest用于区分Gaming * 模组中AfterLoad方法现已移动至加载器完全加载完毕后触发 * 删除了服务器对GameModule的加载,现在只会加载GameModuleServer
44 lines
1.6 KiB
C#
44 lines
1.6 KiB
C#
using System.Net.WebSockets;
|
|
using Milimoe.FunGame.Core.Api.Utility;
|
|
using Milimoe.FunGame.Core.Interface.Base;
|
|
using Milimoe.FunGame.Core.Interface.Sockets;
|
|
using Milimoe.FunGame.Core.Library.Constant;
|
|
using Milimoe.FunGame.Core.Service;
|
|
|
|
namespace Milimoe.FunGame.Core.Library.Common.Network
|
|
{
|
|
public class ClientWebSocket(HTTPListener listener, WebSocket instance, string clientIP, string clientName, Guid token) : IWebSocket, ISocketMessageProcessor
|
|
{
|
|
public HTTPListener Listener => listener;
|
|
public WebSocket Instance => instance;
|
|
public SocketRuntimeType Runtime => SocketRuntimeType.Server;
|
|
public Guid Token => token;
|
|
public string ClientIP => clientIP;
|
|
public string ClientName => clientName;
|
|
public Type InstanceType => typeof(ClientWebSocket);
|
|
|
|
public void Close()
|
|
{
|
|
TaskUtility.NewTask(async () =>
|
|
{
|
|
if (Instance.State == WebSocketState.Open)
|
|
{
|
|
// 安全关闭 WebSocket 连接
|
|
await Instance.CloseAsync(WebSocketCloseStatus.NormalClosure, "服务器正在关闭,断开连接!", CancellationToken.None);
|
|
Listener.ClientSockets.Remove(Token);
|
|
}
|
|
});
|
|
}
|
|
|
|
public SocketResult Send(SocketMessageType type, params object[] objs)
|
|
{
|
|
throw new AsyncSendException();
|
|
}
|
|
|
|
public async Task<SocketResult> SendAsync(SocketMessageType type, params object[] objs)
|
|
{
|
|
return await HTTPManager.Send(Instance, new(type, token, objs));
|
|
}
|
|
}
|
|
}
|